728x90
https://assetstore.unity.com/packages/2d/characters/furball-2d-v1-2-mobile-optimized-40588
FurBall 2D V1.2 mobile optimized | 2D 캐릭터 | Unity Asset Store
Elevate your workflow with the FurBall 2D V1.2 mobile optimized asset from Illustraktion. Find this & more 캐릭터 on the Unity Asset Store.
assetstore.unity.com
귀여워서 이 애셋으로 만들거에요
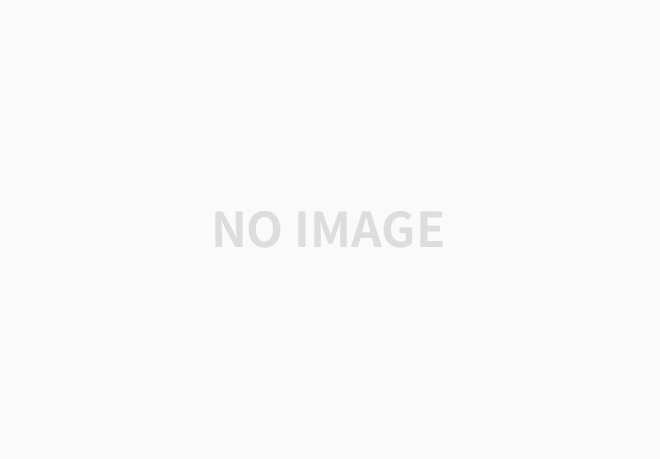
사용할 오브젝트를 꺼내봤어요.
-게임 내용은
발판밟고 올라가서 갇혀있는 친구 구하기
입니다.
- Add 콜라이더
카메라랑 플레이어(날개업는 복실이), 발판만 남기고 다른 오브젝트는 하이라키에서 지워주세요
플레이어와 발판에 2D콜라이더와 ‘Rigidbody 2D’를 추가하세요
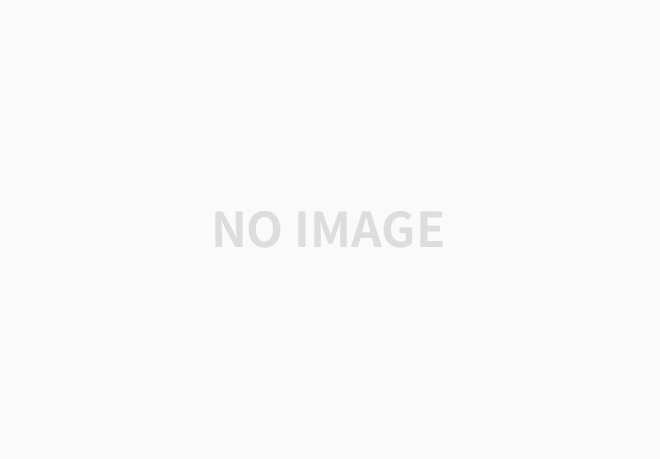
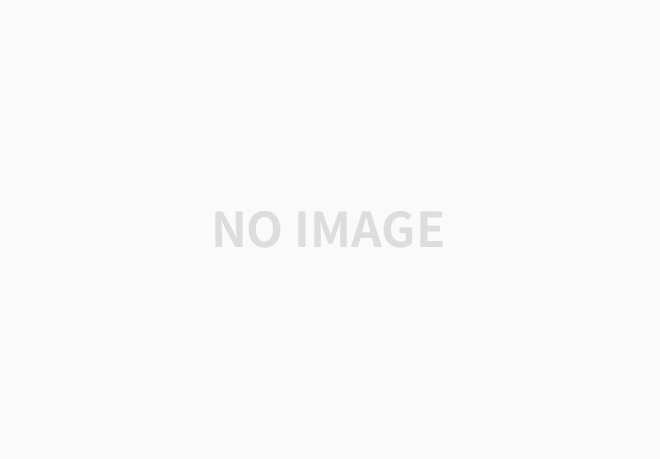
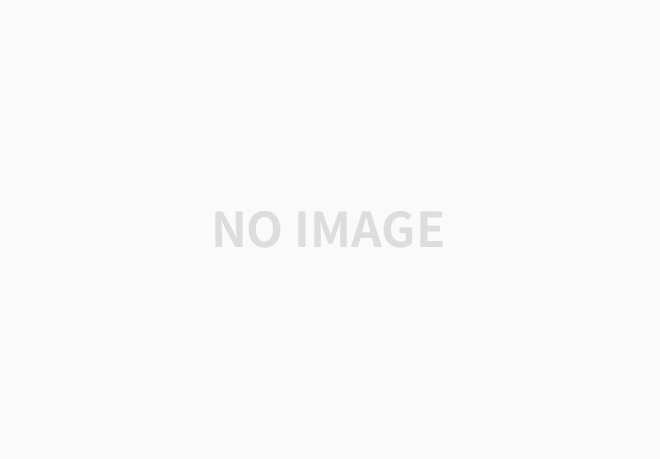
발판의 Rigidbody 2D 수정해주세Body Type을 Kinematic으로 바꾸면 중력에 영향을 받지 않고 그 위치에 머무릅니다.
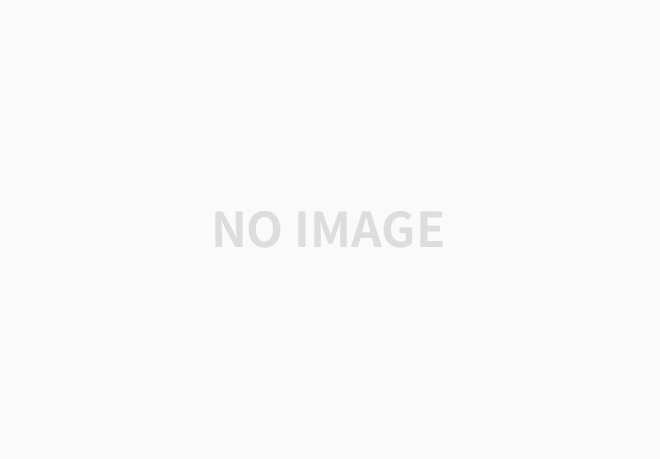
여러번 사용해야할 발판은 프리펩으로 만들어주세요
-플레이어 움직이기
Player 복실이를 움직일 코드를 작성하고 복실이에게 넣어주세요
스크립트 파일명이 겹치지 않게 해주세요. 같은 프로젝트 내에 겹치는 파일명이 있으면 오류가 납니다.
using System.Collections;
using System.Collections.Generic;
using UnityEngine;
public class BallController : MonoBehaviour
{
Rigidbody2D rigid2D;
float jumpForce = 600.0f; // 재생해보고 수정하세요
float walkForce = 30.0f;
float maxWalkSpeed = 2.0f;
// Start is called before the first frame update
void Start()
{
Application.targetFrameRate = 60;
this.rigid2D = GetComponent<Rigidbody2D>();
}
// Update is called once per frame
void Update()
{
// 점프
if (Input.GetKeyDown(KeyCode.Space))
{
this.rigid2D.AddForce(transform.up * this.jumpForce);
}
// 좌우 이동
int key = 0;
if (Input.GetKey(KeyCode.RightArrow)) key = 1;
if (Input.GetKey(KeyCode.LeftArrow)) key = -1;
// 플레이어의 속도
float speedx = Mathf.Abs(this.rigid2D.velocity.x);
// Mathf
// 사용방법 : struct UnityEngine.Mathf
// A collection of common math functions. 일반적인 수학 함수 모음입니다.
// Mathf.Ab
// 사용 : float Mathf.Abs(float f)
// Returns the absolute value of f. f의 절대값을 반환합니다.
// velocity
// 사용 : Vector2 Rigidbody2D.velocity { get; set;}
// Linear velocity of the Rigidbody in units per second.
// 초당 단위로 표시되는 강체의 선형 속도입니다.
// 스피드 제한
if (speedx < this.maxWalkSpeed)
{
this.rigid2D.AddForce(transform.right * key * this.walkForce);
}
}
}
플래이하고 움직여보세요
복실이가 데굴데굴 굴러가네요 ㅎㅎㅎ
이걸 막기 위해 리기드바디에서 z축 회전을 막을게요
(하이라키)Player > (Inspect)Rigidbody 2D > Constraints > Freeze Rotation > Z 체크
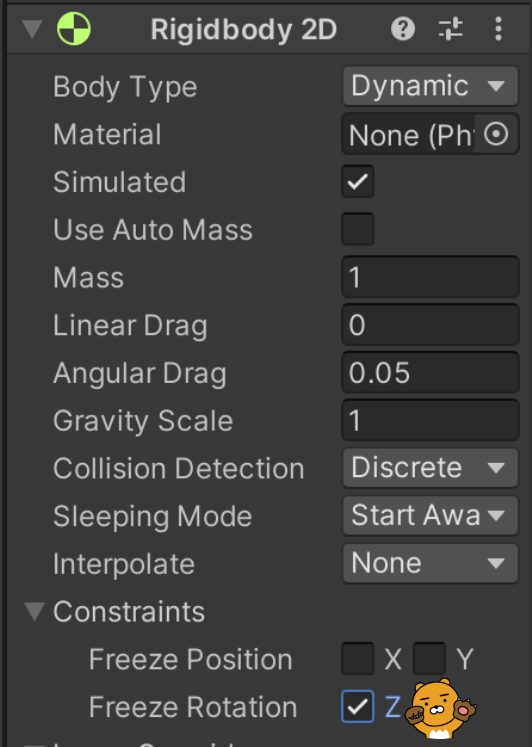
-플레이어 이동방향에 따라 좌우 반전 시키기
플레이어가 잘 움직이나요?
이번에는 플레이어 이동방향에 따라 자동으로 좌우반전 시킬게요.
BallController.cs 에 아래 코드를 추가합니다.
// 움직이는 방향에 따라 좌우 반전
if (key != 0)
{
transform.localScale = new Vector3(key, 0, 0);
// transform.localScale : x축 크기를 1,-1로 설정하여 좌우 반전이 되도록 합니다
}
전체코드
더보기
using System.Collections;
using System.Collections.Generic;
using UnityEngine;
public class BallController : MonoBehaviour
{
Rigidbody2D rigid2D;
float jumpForce = 600.0f; // 재생해보고 수정하세요
float walkForce = 30.0f;
float maxWalkSpeed = 2.0f;
// Start is called before the first frame update
void Start()
{
Application.targetFrameRate = 60;
rigid2D = GetComponent<Rigidbody2D>();
}
// Update is called once per frame
void Update()
{
// 점프
if (Input.GetKeyDown(KeyCode.Space))
{
rigid2D.AddForce(transform.up * jumpForce);
}
// 좌우 이동
int key = 0;
if (Input.GetKey(KeyCode.RightArrow)) key = 1;
if (Input.GetKey(KeyCode.LeftArrow)) key = -1;
// 플레이어의 속도
float speedx = Mathf.Abs(this.rigid2D.velocity.x);
// Mathf
// 사용방법 : struct UnityEngine.Mathf
// A collection of common math functions. 일반적인 수학 함수 모음입니다.
// Mathf.Ab
// 사용 : float Mathf.Abs(float f)
// Returns the absolute value of f. f의 절대값을 반환합니다.
// velocity
// 사용 : Vector2 Rigidbody2D.velocity { get; set;}
// Linear velocity of the Rigidbody in units per second.
// 초당 단위로 표시되는 강체의 선형 속도입니다.
// 스피드 제한
if (speedx < maxWalkSpeed)
{
rigid2D.AddForce(transform.right * key * walkForce);
}
// 움직이는 방향에 따라 좌우 반전
if (key != 0)
{
transform.localScale = new Vector3(0.4f * key, 0.4f, 0.4f);
// transform.localScale : x축 크기에 1,-1를 곱하여 좌우 반전이 되도록 합니다
}
}
}
발판을 적당하게 배치해 주세요,
플래이해보고 jumpForce 와 발판 위치, 크기를 조절하세요

밖으로 떨어지는게 싫다면 양옆을 막는 방법도 있어요(난이도가 쉬워져요)
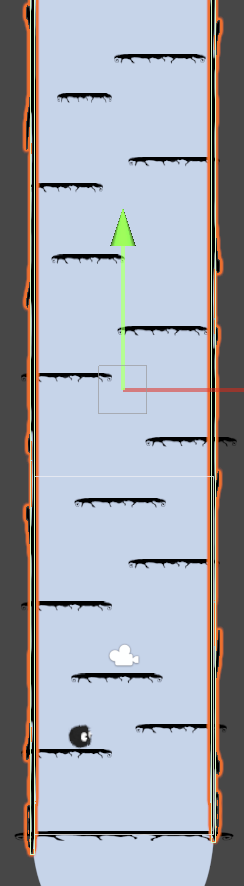
카메라를 캐릭터따라 움직이게 하기
카메라 스크립트를 작성합니다.
using System.Collections;
using System.Collections.Generic;
using UnityEngine;
public class BallCamera : MonoBehaviour
{
GameObject player;
void Start()
{
// "Player"라는 이름을 가진 게임 오브젝트를 찾아 player 변수에 할당
this.player = GameObject.Find("Player");
}
void Update()
{
// 플레이어의 위치를 가져옴
Vector3 playerPos = this.player.transform.position;
// 카메라의 위치를 업데이트하여 y축만 플레이어의 y축 위치와 일치하도록 함
transform.position = new Vector3(
transform.position.x, playerPos.y, transform.position.z);
}
}
카메라에 스크립트를 적용하세요
플래이하면 카메라가 따라오는걸 볼 수 있습니다.
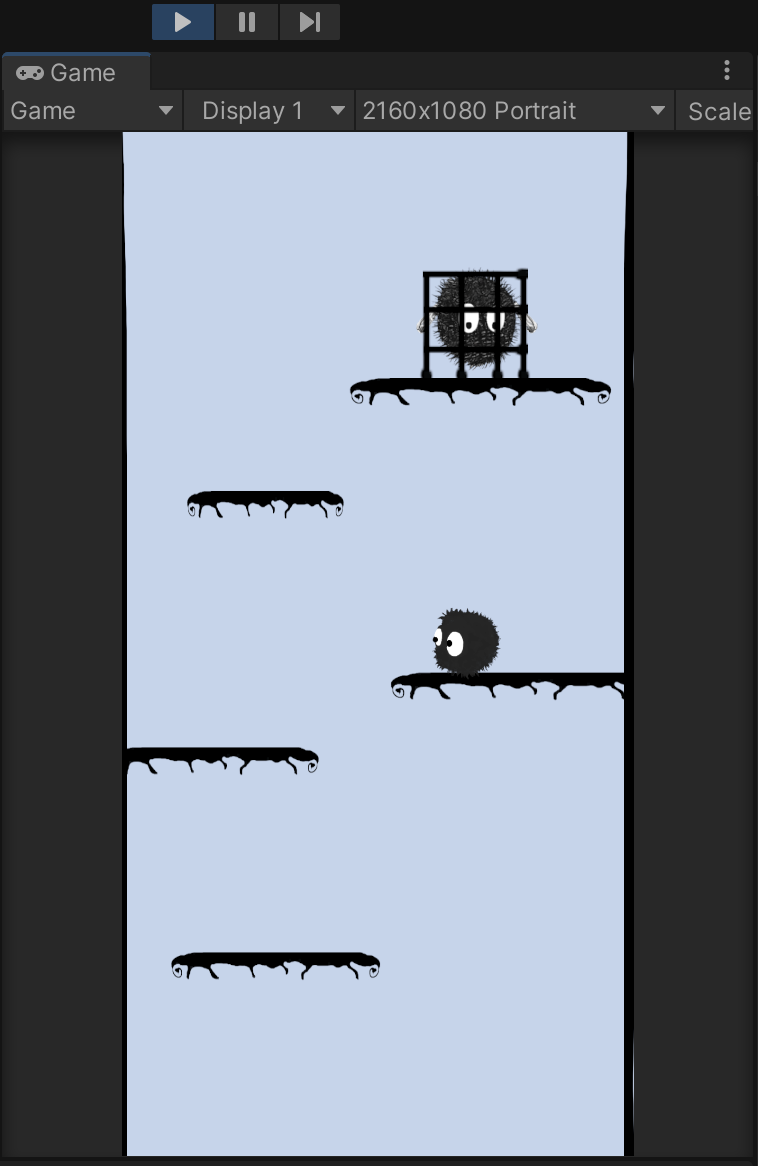
-종료씬
게임이 종료되면(친구를 구하면) 띄울 Scene를 만들어 주세요.
Project 창에 서 우클릭 > Creats > Scene


빈 오브젝트를 만들어(ClearDirector) 아래 스크립트를 넣어주세요
using System.Collections;
using System.Collections.Generic;
using UnityEngine;
using UnityEngine.SceneManagement;// 씬 전환 시 필수
public class ClearDirector : MonoBehaviour
{ // 종료씬 -> 게임"
void Update()
{
if (Input.GetMouseButtonDown(0))
{
SceneManager.LoadScene("FurBall"); // 게임씬의 이름을 넣으세요
}
}
}
-
Builld Setting에 종료씬과 플레이씬을 넣어주세요
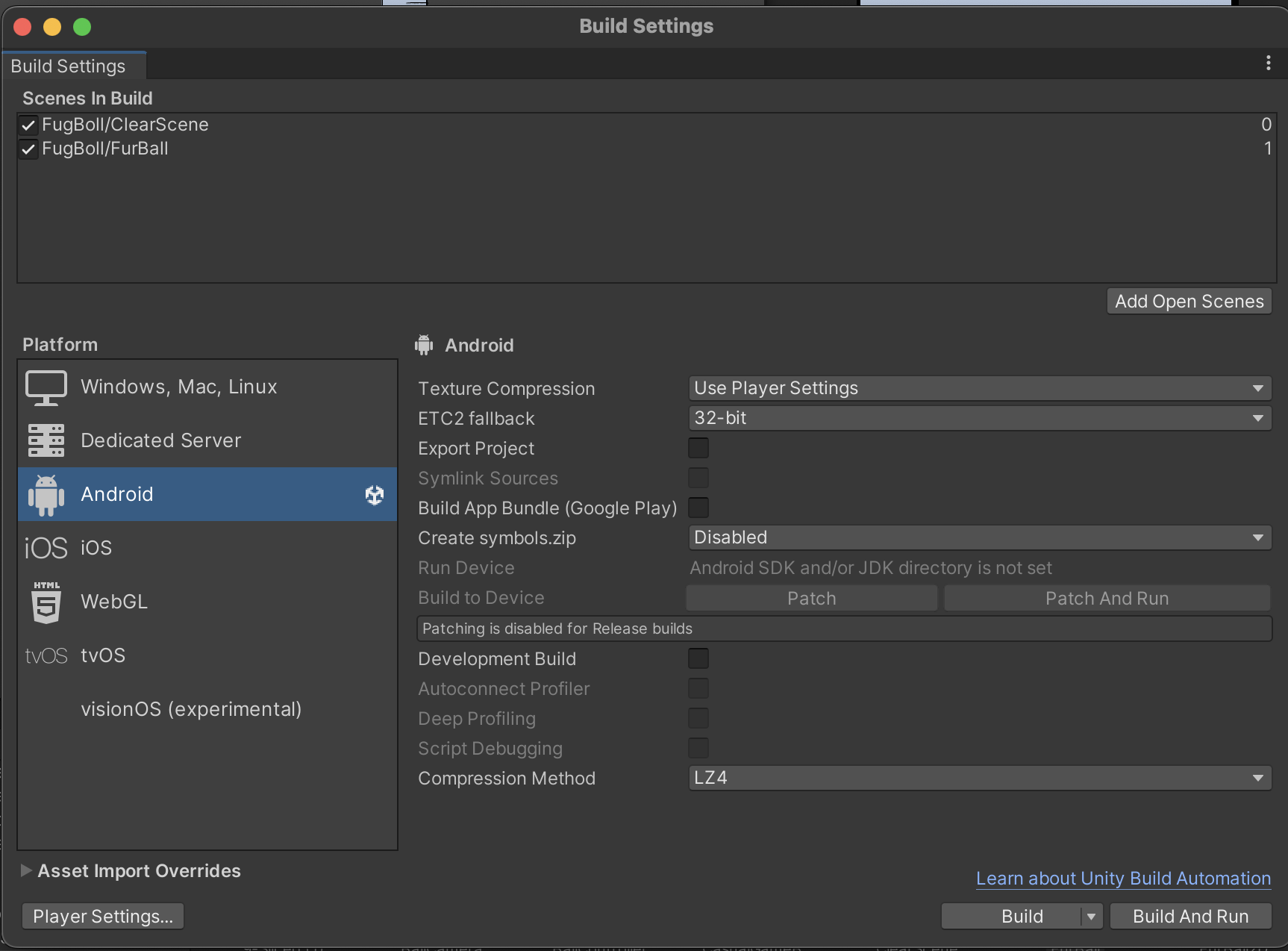
친구를 구하면 게임종료
맨 윗칸에 날개달린 친구를 적당히 가둬주세요
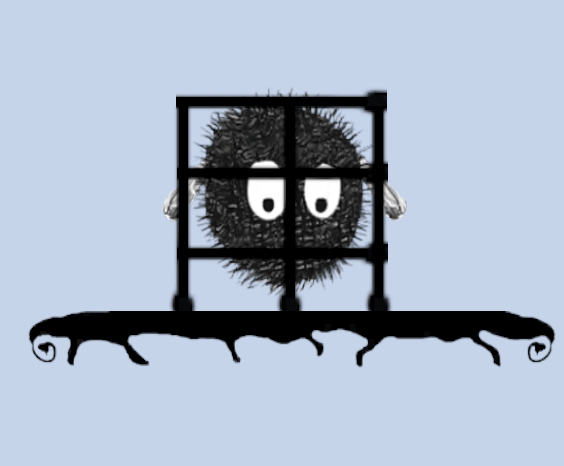
플레이어가 감옥(?)에 도달하는걸 감지하기 위해 Box Collider 2D를 감옥을 구성하는 막대에 넣고 is Trigger도 체크해주세요
(하나에만 넣어도 되요. 전 가로하단막대에만 넣었습니다.)
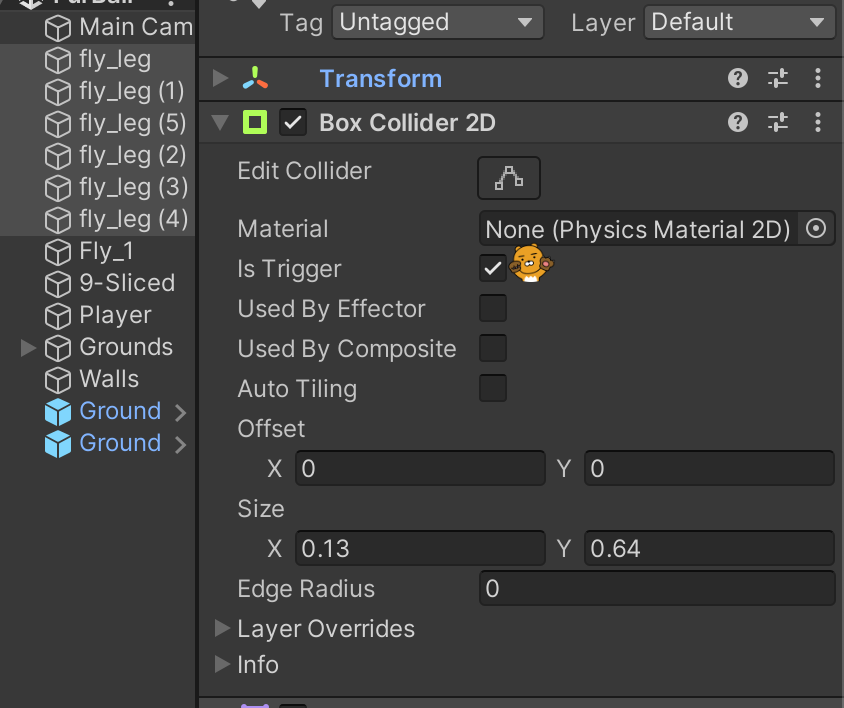
BallController.cs에 아래 코드를 추가하세요(Update나 Start 밖에 추가하세요)
// 끝 도착
// 게임 -> 종료 씬
void OnTriggerEnter2D(Collider2D collision)
{
SceneManager.LoadScene("ClearScene"); // 게임씬의 이름을 넣으세요
}
https://youtube.com/shorts/goMDNsGd_BU?feature=share
// 애니메이터 추가, 점프제한, 화면밖으로 나가면 재시작 추가하기
코드
https://github.com/BlueStrobus/UnityCsCode/tree/main/%E1%84%87%E1%85%A9%E1%86%A8%E1%84%89%E1%85%B5%E1%86%AF%E1%84%8B%E1%85%B5%E1%84%8E%E1%85%B5%E1%86%AB%E1%84%80%E1%85%AE%E1%84%90%E1%85%A1%E1%86%AF%E1%84%8E%E1%85%AE%E1%86%AF%E1%84%89%E1%85%B5%E1%84%8F%E1%85%B5%E1%84%80%E1%85%B5
'UNITY > Unity Study' 카테고리의 다른 글
큐브 옮기기 게임 - Unity 3D (3) | 2023.09.06 |
---|---|
시간내에 목적지 도달하기 (0) | 2023.08.30 |
화살피하기 Unity 2D (0) | 2023.08.22 |
목적지까지 자동차 움직이기 Unity (0) | 2023.08.21 |
C# (0) | 2023.08.21 |